String to Integer (atoi)
Implement atoi which converts a string to an integer.
(字符串转32位整形)
Note:
Assume we are dealing with an environment which could only store integers within the 32-bit signed integer range: \([−2^{31}, 2^{31} − 1]\). For the purpose of this problem, assume that your function returns 0 when the reversed integer overflows.
Example:
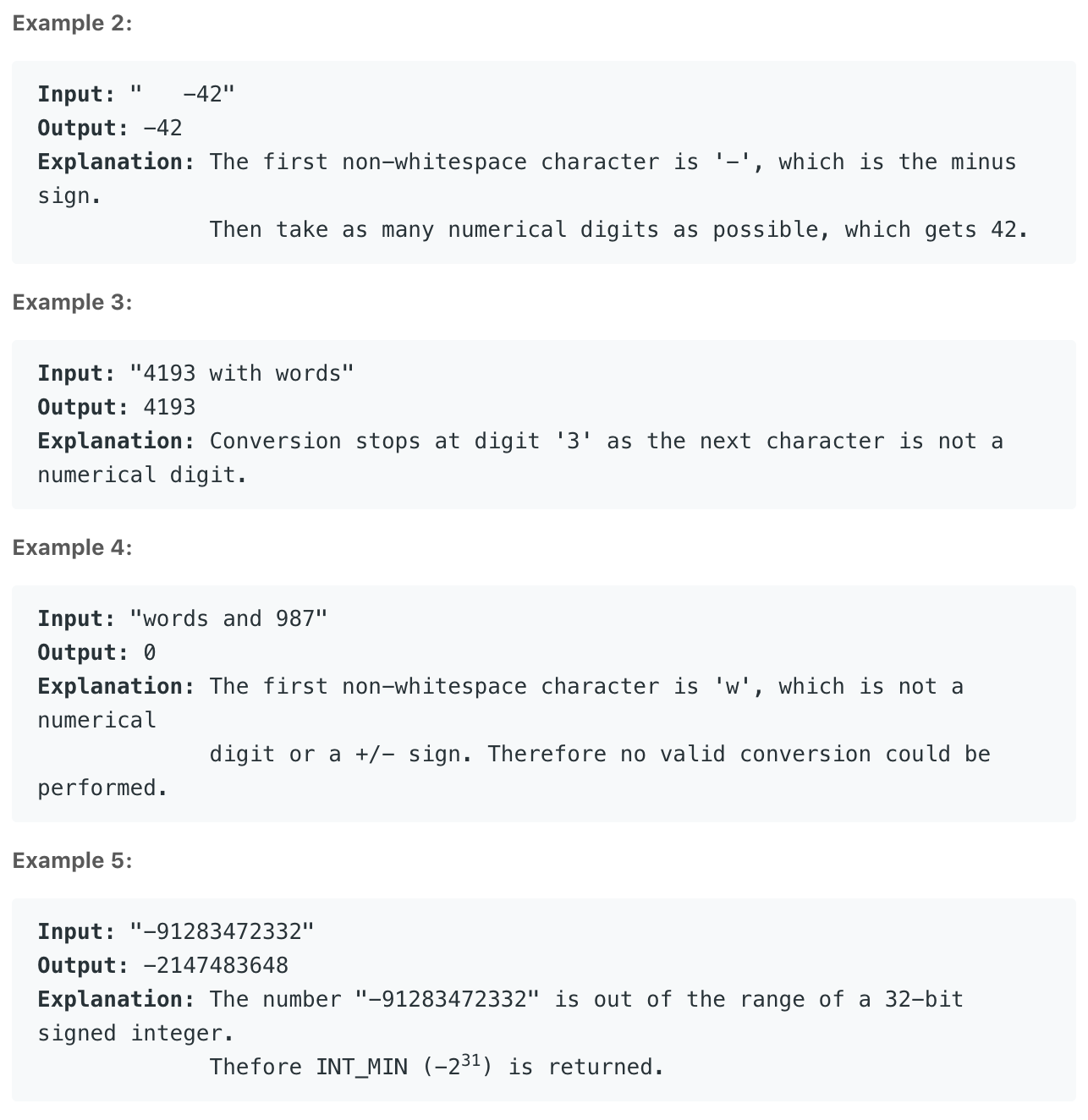
字符串过滤-正则表达式
首先删除字符串首尾的空格,通过正则表达式过滤剩下以 “+-0123456789” 开头的句子,提取剩下的字符串首部的数字串,再将其转换为整形。(注意需要将超出范围的部分返回0。)
1 | import re |
注:需要考虑 \(+/- \) 出现在数字字符串中间的部分,因此 ‘0-1’ 需要通过if i != 0 and (char == '+' or char == '-')
过滤掉。当然,也可以直接通过match的group()函数,其返回值为匹配的字符串,进而获取开始的数值字符串。
1 | num_string = re.match(r'^(\-|\+)?\d+', string).group() |