Letter Combinations of a Phone Number
Given a string containing digits from 2-9 inclusive, return all possible letter combinations that the number could represent. A mapping of digit to letters (just like on the telephone buttons) is given below. Note that 1 does not map to any letters.
(根据九宫格键盘将数字映射到字符)
Example:
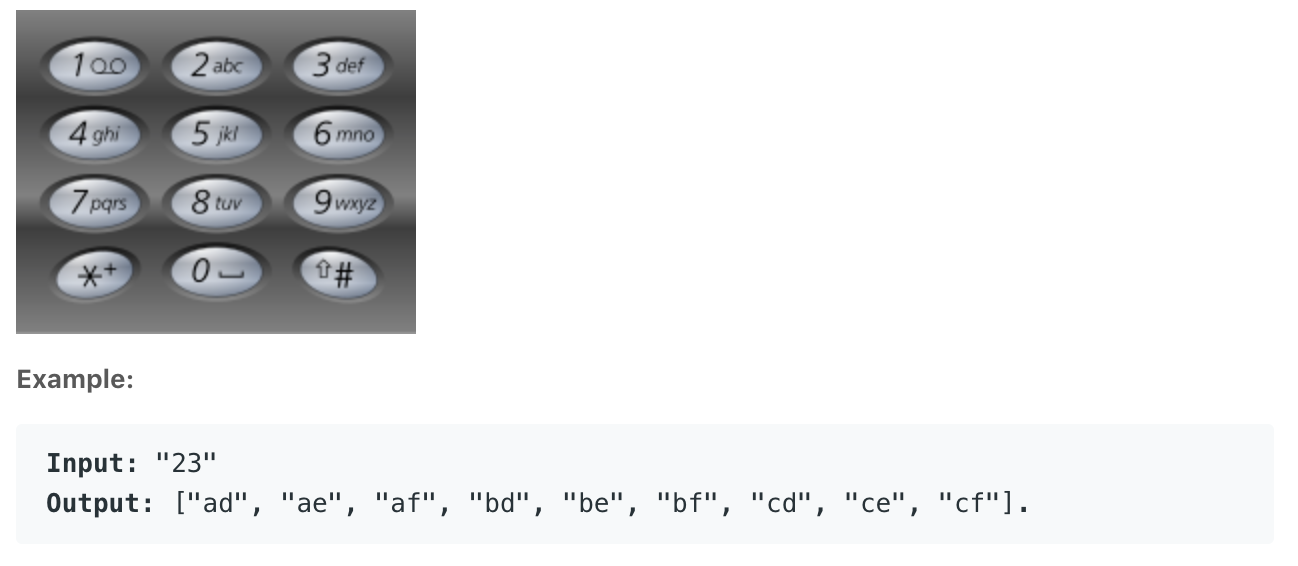
1. map / hash_table 进行索引
这个问题很直观就是建立一个map进行索引。在提交过程中,发现如果考虑了digits == ''
的情况,时间大幅度降低。说明在这种测试情况下,多考虑一些极端情况,直接返回结果,会使得整体的测试时间减少很多。
1 | class Solution: |