Valid Parentheses
Given a string containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid.
(判断有效的括号对)
An input string is valid if:
- Open brackets must be closed by the same type of brackets.
- Open brackets must be closed in the correct order.
Note that an empty string is also considered valid.
Example:
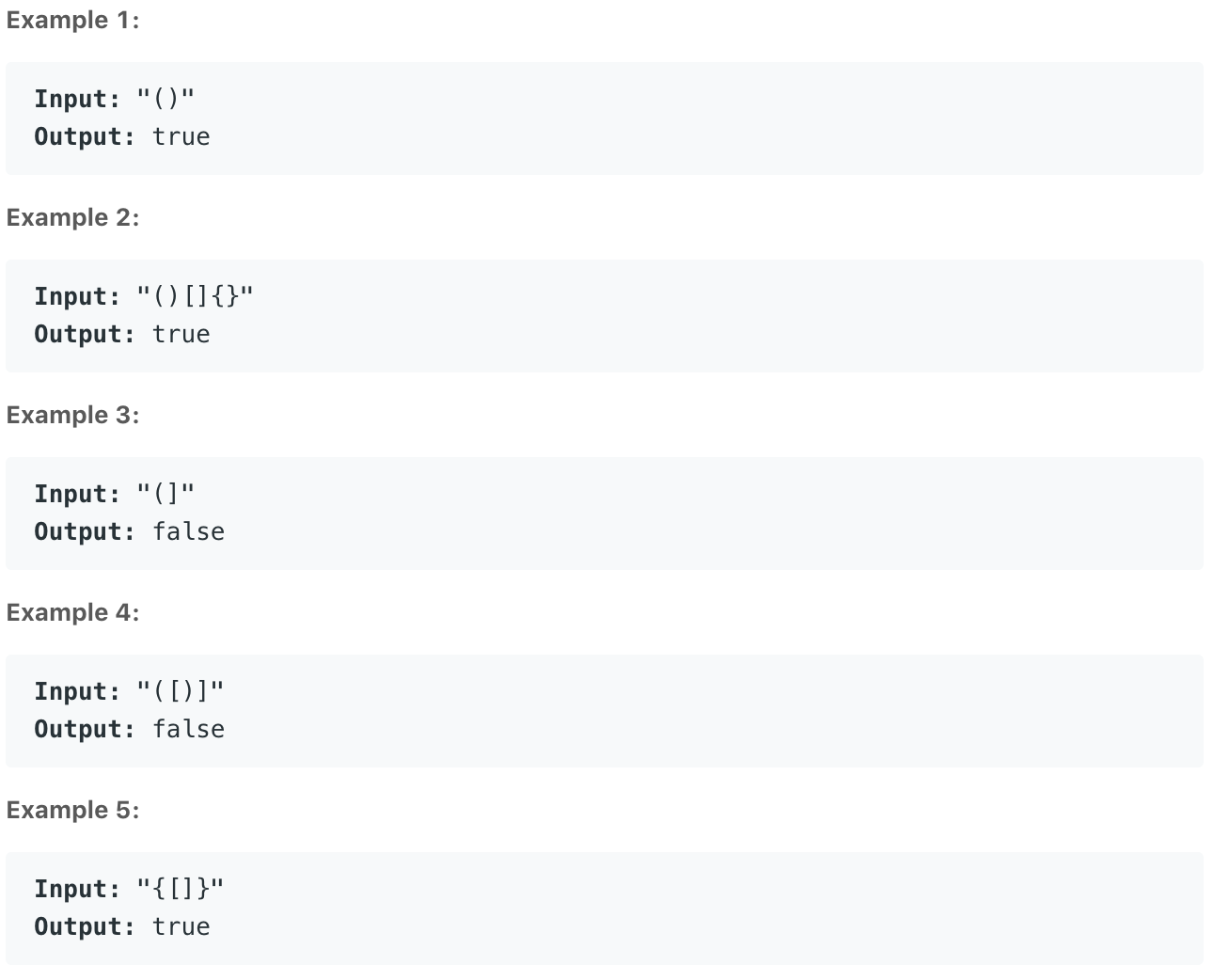
1. 栈匹配
括号的匹配问题是一个很直观的栈的应用问题。具体实现过程如下:
1 | class Solution: |
2. 栈匹配
后来看别人的解答过程中有一种更直观简单的栈匹配过程。具体实现过程如下:
1 | class Solution: |