Merge k Sorted Lists
Merge k sorted linked lists and return it as one sorted list. Analyze and describe its complexity.
(合并k个有序链表)
Example:
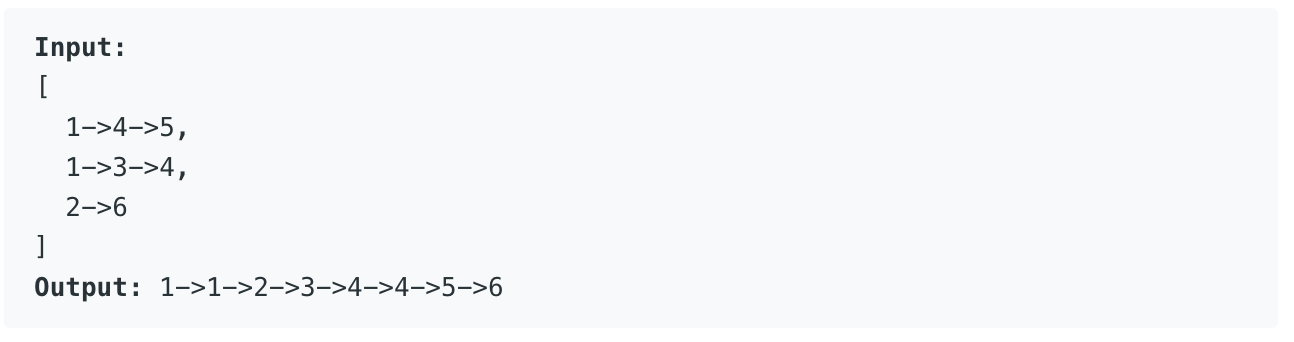
1. 分治法
这个问题是一个典型的分治法解决的问题。其时间复杂度为 \(O(mlog(n))\),其中 \(n\) 为序列个数,\(m\) 为所有序列的长度和。具体实现过程如下:
1 | class Solution: |
2. 堆排序
另外一种方式是遍历所有的链表,并用堆保存并排序,然后根据堆构建最终的链表。其时间复杂度为 \(O(mlog(n))\),其中 \(n\) 为序列个数,\(m\) 为所有序列的长度和。具体实现过程如下:
1 | from heapq import heappush, heappop |