Sudoku Solver
Write a program to solve a Sudoku puzzle by filling the empty cells.
(求解数独盘)
A sudoku solution must satisfy all of the following rules:
- Each of the digits 1-9 must occur exactly once in each row.
- Each of the digits 1-9 must occur exactly once in each column.
- Each of the the digits 1-9 must occur exactly once in each of the 9
3x3
sub-boxes of the grid. - Empty cells are indicated by the character ‘.’.
Note:
- The given board contain only digits 1-9 and the character ‘.’.
- You may assume that the given Sudoku puzzle will have a single unique solution.
- The given board size is always 9x9.
Example:
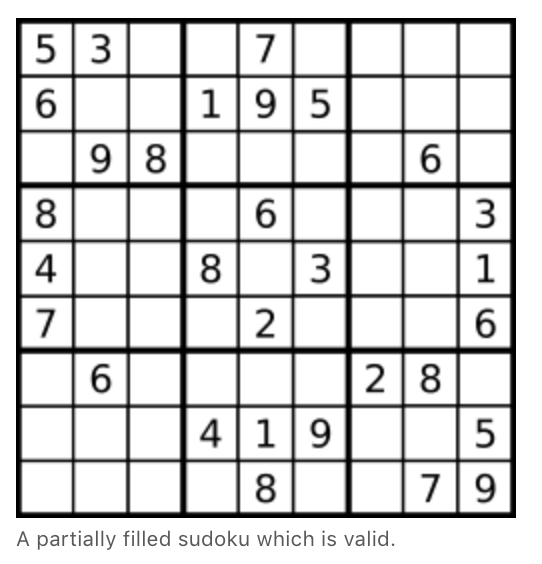
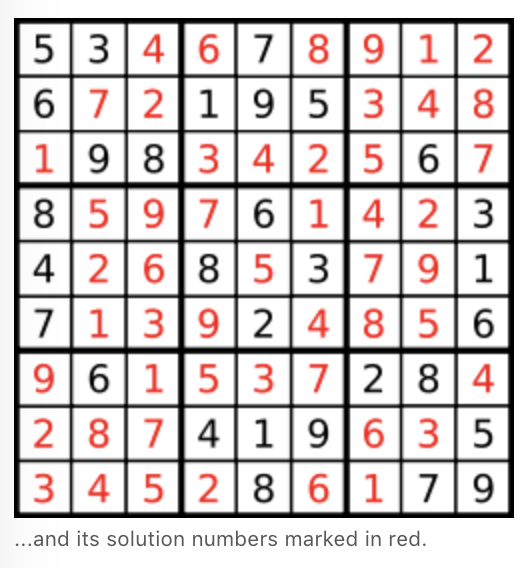
1. 回溯法 Back Tracking
求解数独、八皇后等等都是一个很经典的应用回溯法(深度优先搜索 + 剪枝)的例子。主要分为三个部分:
- 寻找下一层节点,此处为find_next_empty()。
- 判断限制条件,此处为check_constraint()。
- 针对找到的节点继续进行 DFS, 如果已经满足要求则返回True,否则继续判断并不满足要求则回溯,此处为back_track()。
1 | class Solution: |