Valid Sudoku
Determine if a 9x9 Sudoku board is valid. Only the filled cells need to be validated according to the following rules.
(数独盘有效性判断)
- Each row must contain the digits 1-9 without repetition.
- Each column must contain the digits 1-9 without repetition.
- Each of the 9
3x3
sub-boxes of the grid must contain the digits 1-9 without repetition.
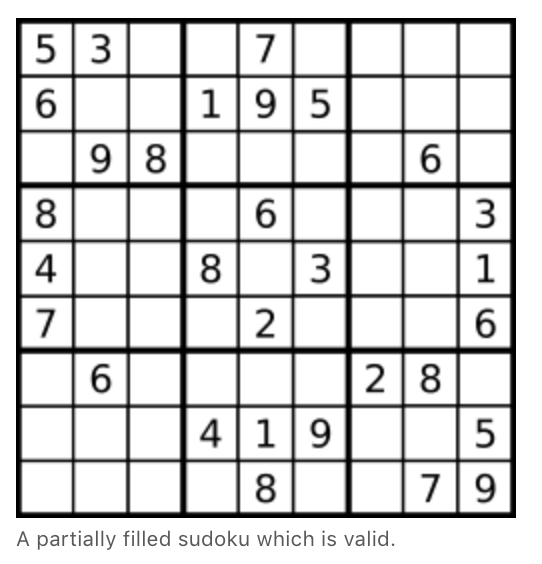
Note:
- A Sudoku board (partially filled) could be valid but is not necessarily solvable.
- Only the filled cells need to be validated according to the mentioned rules.
- The given board contain only digits 1-9 and the character ‘.’.
- The given board size is always 9x9.
Example:
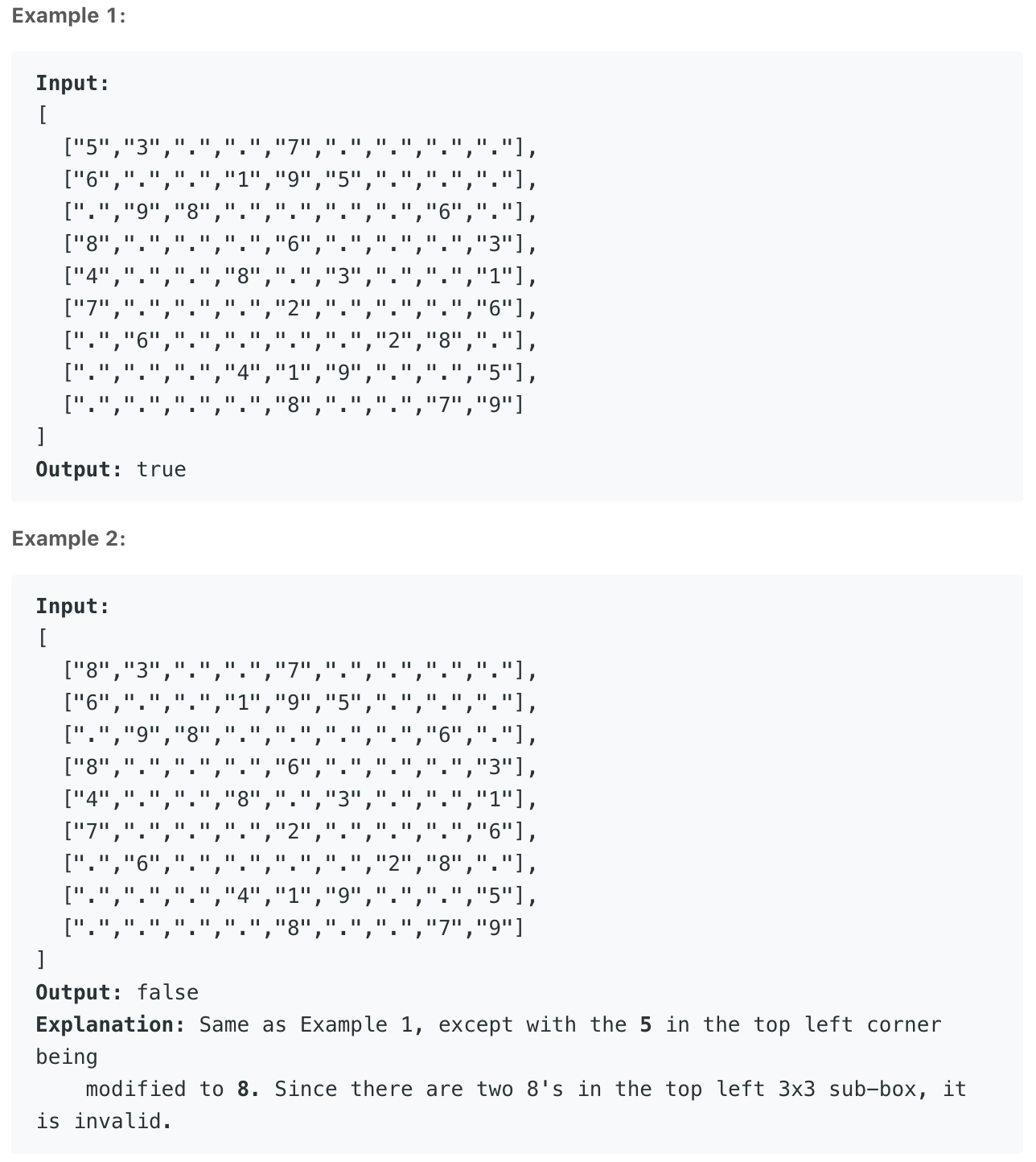
1. 依次check
根据题中设定的要求逐一排查,一旦检测到不符合标准的部分就返回 False。
1 | class Solution: |
2. 使用Hash Map
只需要遍历一次矩阵,在遍历过程中保存每个的数值的行、列的index,并保存数值属于9个正方形中的哪一个(i//3, j//3)。具体实现过程如下:
1 | class Solution: |