Combination Sum II
Given a collection of candidate numbers (candidates) and a target number (target), find all unique combinations in candidates where the candidate numbers sums to target. Each number in candidates may only be used once in the combination.
(从集合中挑选和为特定值的数字组合,同一元素只可选一次)
Note:
- All numbers (including target) will be positive integers.
- The solution set must not contain duplicate combinations.
Example:
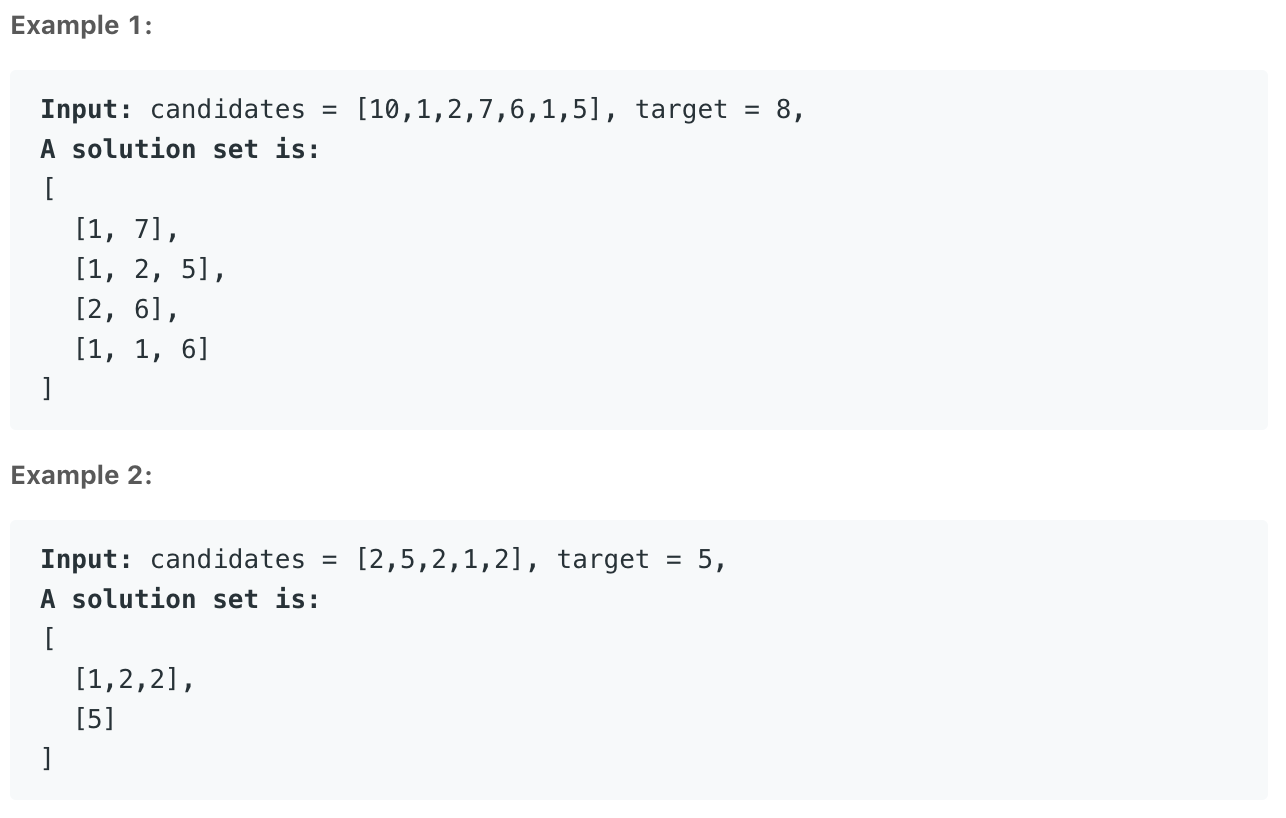
1. 回溯法
这道题与上一题的区别在于:
- 这个题限制了 candidates 中的元素只可以用一次。因此将遍历后的数组 candidates[i+1:] 继续遍历(因为candidates已经排序过了)。
- candidates 可能存在有重复的元素。因此在生成最终的结果时,需要查重 list(re_set) not in self.result。
1 | class Solution: |