First Missing Positive
Given an unsorted integer array, find the smallest missing positive integer.
(在未排序数组中找到最小的非正数)
Note:
- Your algorithm should run in O(n) time and uses constant extra space.
Example:
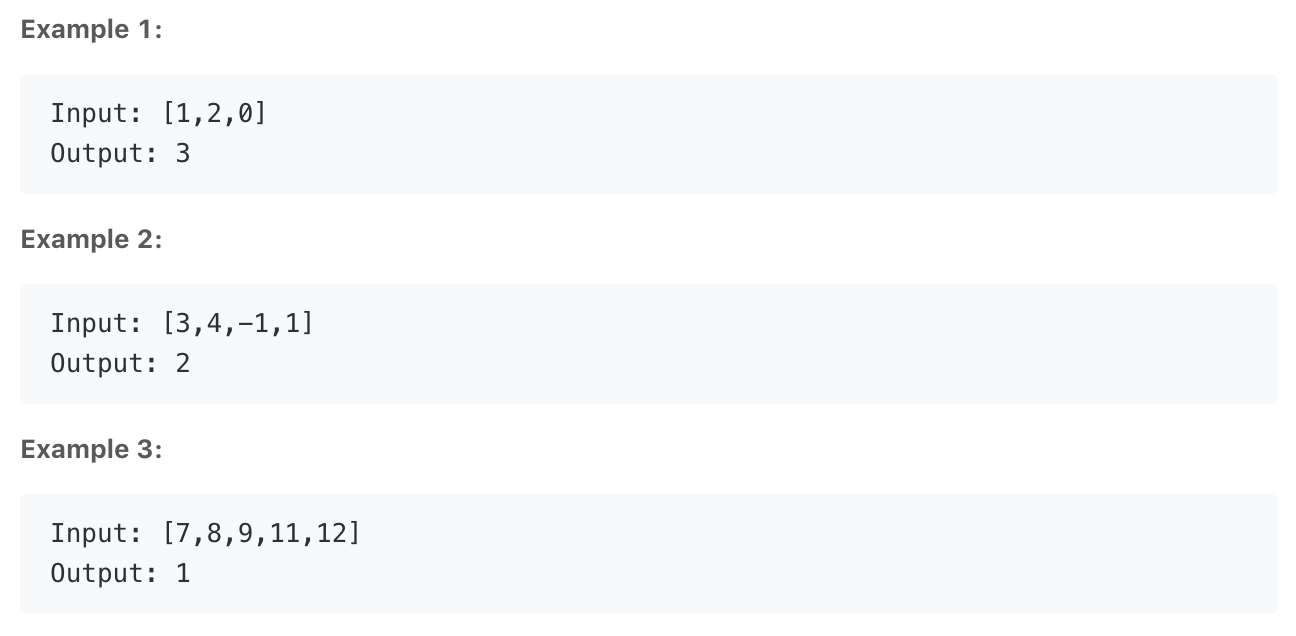
1. 哈希法
要在时间复杂度为 \( O(n)\),且常数额外空间的情况下完成这个问题。哈希法的思路是分析数组中的正数应该在的位置。我们发现例子中的 [3, 4, -1, 1] 的答案是2,我们可以通过遍历数组将其转换为 [1, -1, 3, 4],即数组中的index为 i 的值应该是正数 i + 1,从左向右遍历若不满足这个要求,则这就是我们要找的缺失的最小正数。
1 | class Solution: |