Multiply Strings
Given two non-negative integers num1 and num2 represented as strings, return the product of num1 and num2, also represented as a string.
(用非库函数的方式返回两个字符串表示的整数的乘积)
Example:
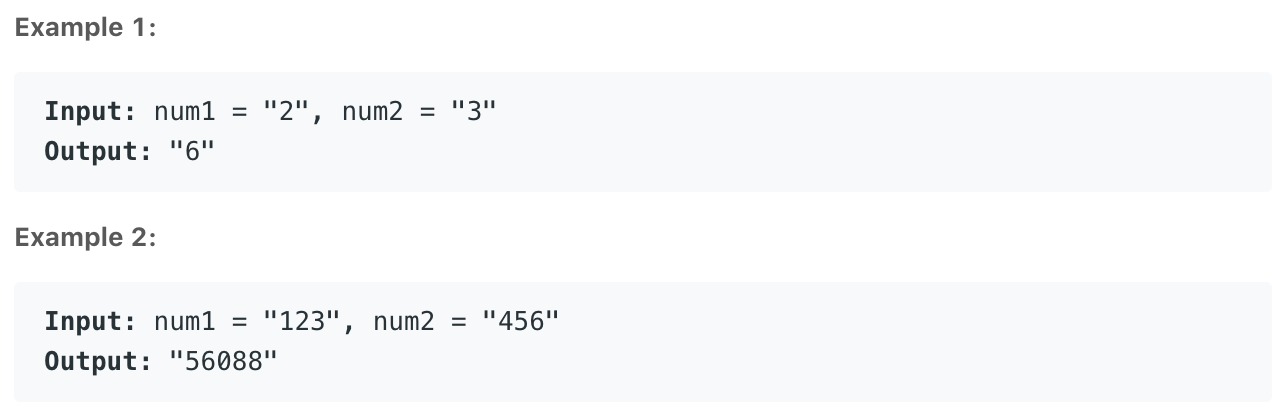
Note:
- The length of both num1 and num2 is < 110.
- Both num1 and num2 contain only digits 0-9.
- Both num1 and num2 do not contain any leading zero, except the number 0 itself.
- You must not use any built-in BigInteger library or convert the inputs to integer directly.
实现库函数 atoi 和 itoa
由于题中备注了不让使用自带的库函数,因此可以自己实现。其中在 python 中 ord()
表示将字符转换为ASCII码,chr()
表示将ASCII码转换为字符。具体实现过程如下:
1 | class Solution: |