Insert Interval
Given a set of non-overlapping intervals, insert a new interval into the intervals (merge if necessary). You may assume that the intervals were initially sorted according to their start times.
(插入间隔区间)
Example:
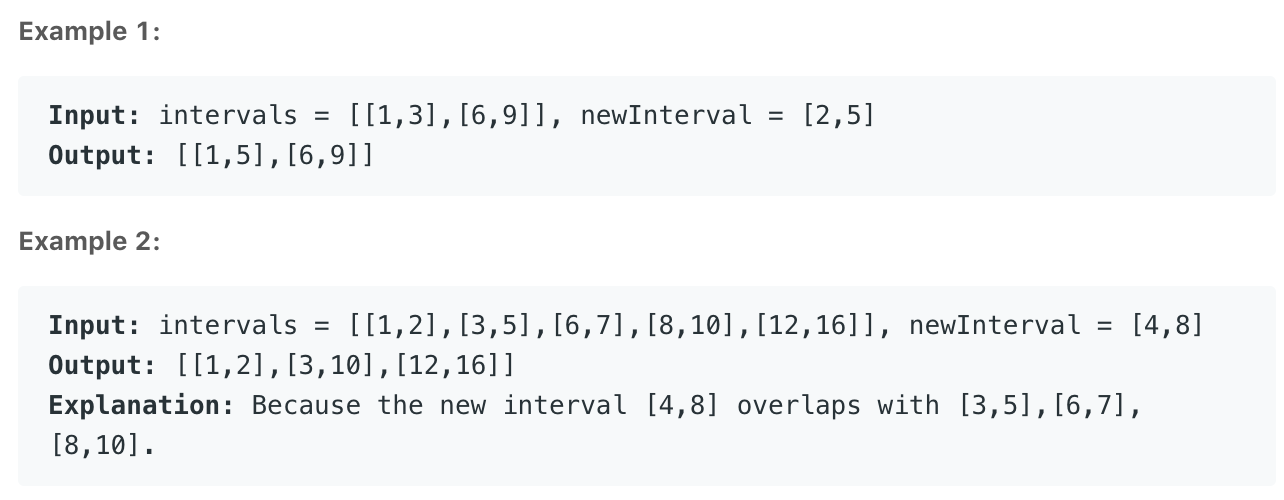
1. 遍历间隔区间
直接遍历间隔区间数组,并在遇到交叉的情况下时不断更新新插入的间隔区间的起止位置。具体实现过程如下:
1 | # Definition for an interval. |
2. 合并间隔区间
借用前一道题目的方法,把待插入的间隔区间和数组合并,然后合并交叉的区间。具体实现方法如下:
1 | # Definition for an interval. |