Text Justification
(文本左右对齐)
Note:
- A word is defined as a character sequence consisting of non-space characters only.
- Each word’s length is guaranteed to be greater than 0 and not exceed maxWidth.
- The input array words contains at least one word.
Example:
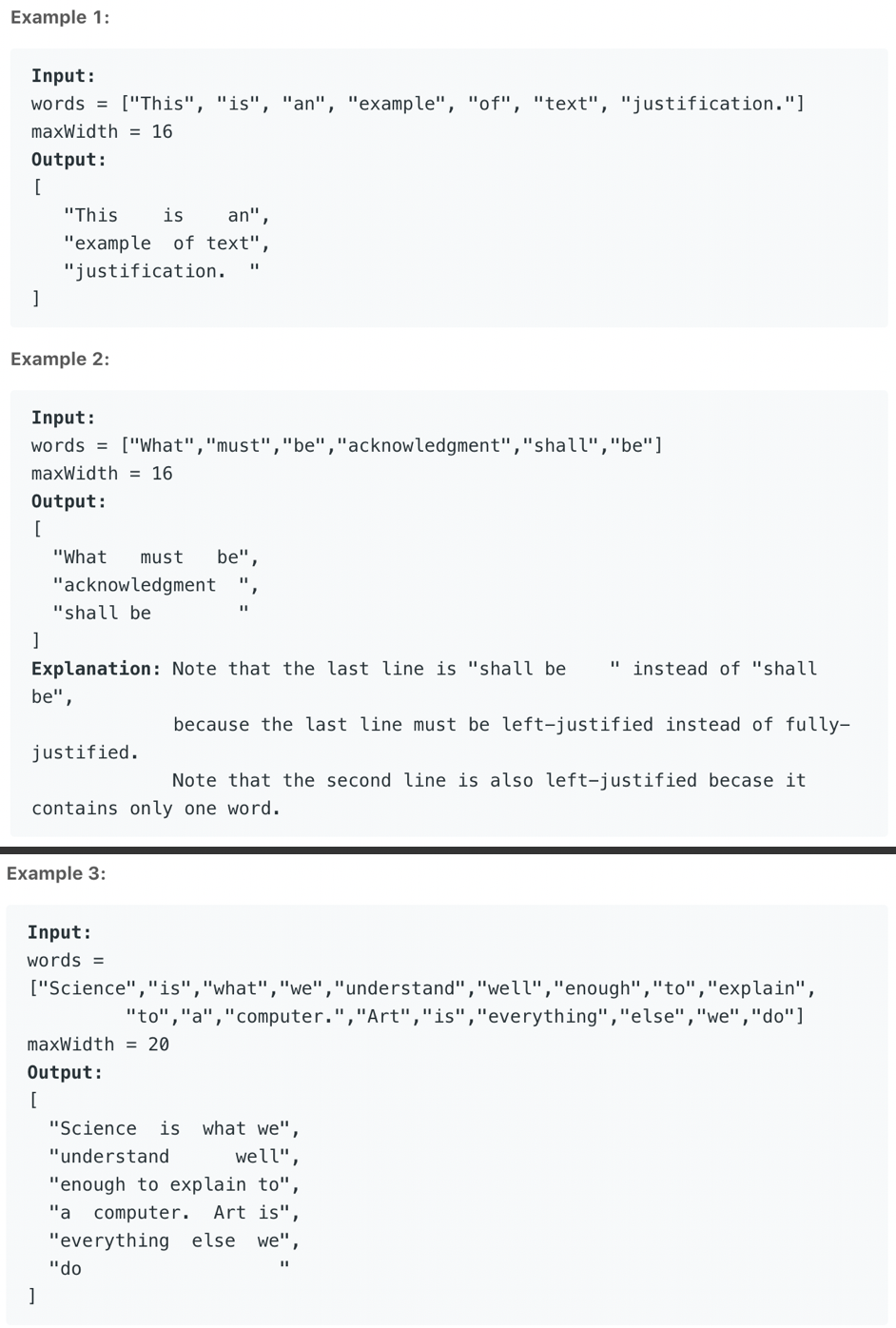
字符串遍历
具体考虑分析需要分为以下几步:
- 计算每行最多显示单词的数量,记录起始单词位置(结束指针单词不包含在内),这里要注意,单词与单词之间的都要求有空格,所以除了开始的单词,每个单词的长度还要加1
- 计算显示每句话需要的空格的数量
- 计算每句话中单词之间需要均匀填充的空格数量。这里要注意最后一行不需要进行均匀填充,不能均匀填充的空格数量要记录下来,优先分给左边的单词间空格
- 按照之前计算好的空格数量将单词连接成语句。
- 要考虑到最后一句是在最右端填充所有的空格
1 | class Solution(object): |