Search A 2D Matrix
Write an efficient algorithm that searches for a value in an m x n matrix. This matrix has the following properties: Integers in each row are sorted from left to right. The first integer of each row is greater than the last integer of the previous row.
(查找数值是否在有序矩阵中)
Example:
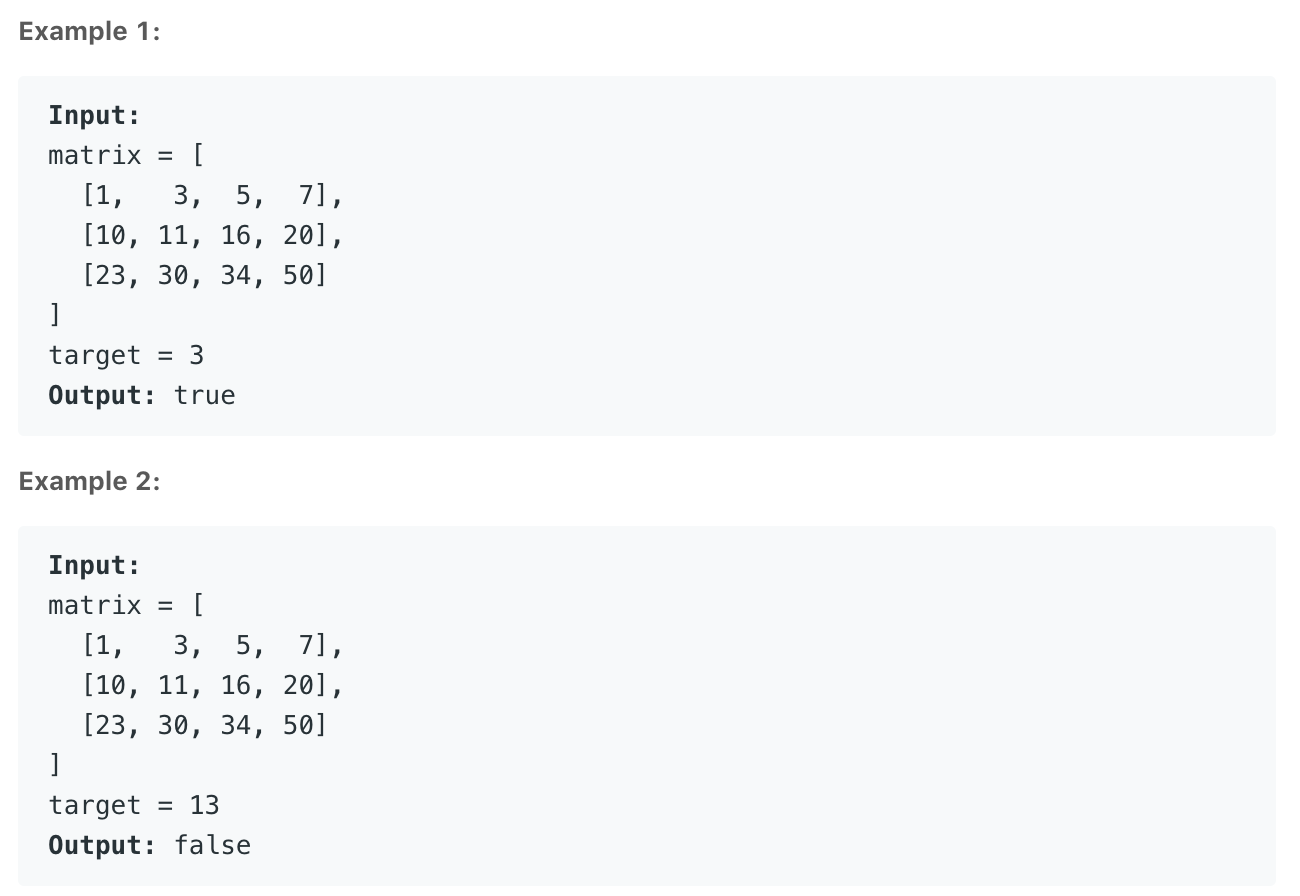
1. 二分查找
1 | class Solution: |
Note: 注意矩阵为空的情况哦~