Remove Duplicates from Sorted Array II
Given a sorted array nums, remove the duplicates in-place such that duplicates appeared at most twice and return the new length. Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory.
(删除数组中超过两次的元素,in-place, 限制空间复杂度)
Example:
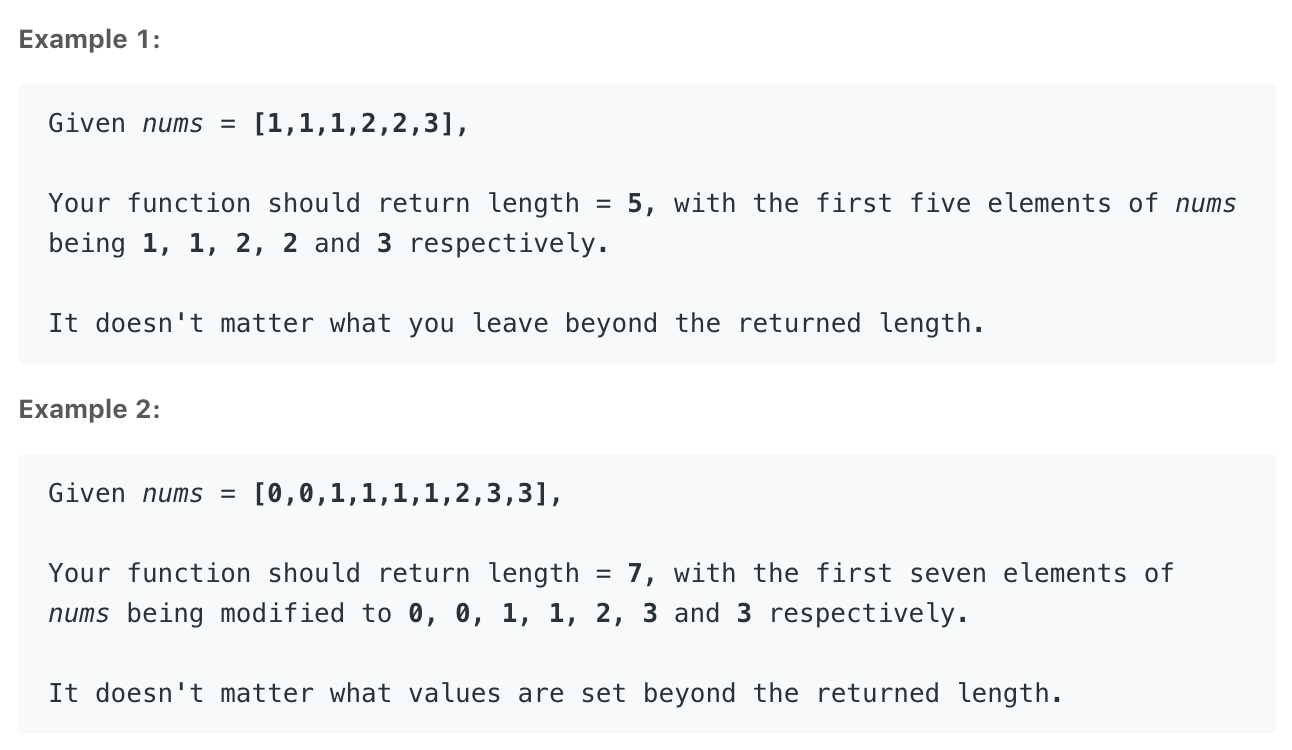
1. 重复元素个数
需要维护一个变量来记录在遍历过程中数字是重复次数k,以及元素需要前移的步数move_before。具体实现过程如下:
1 | class Solution: |