Binary Tree Maximum Path Sum
Given a non-empty binary tree, find the maximum path sum. For this problem, a path is defined as any sequence of nodes from some starting node to any node in the tree along the parent-child connections. The path must contain at least one node and does not need to go through the root.
(二叉树最大路径和)
Example:
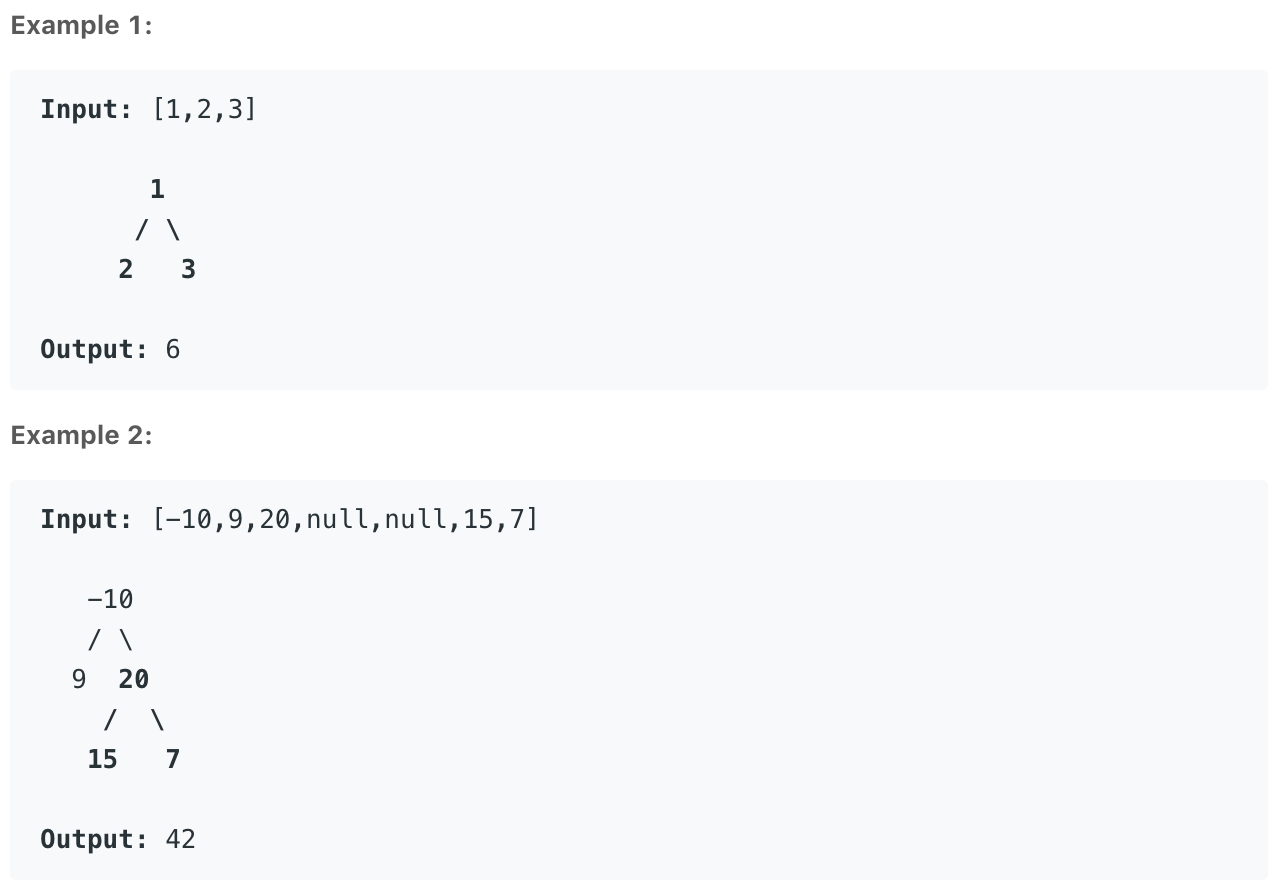
1. 递归
在二叉树中找一条路径,使得该路径的和最大。该路径可以从二叉树任何结点开始,也可以到任何结点结束。因此在递归求一条经过root的最大路径时,这条路径可能是:
- 左边某条路径 + root + 右边某条路径
- root + 左边某条路径
- root + 右边某条路径
- root
具体实现过程如下:
1 | # Definition for a binary tree node. |