Word Ladder
Given two words (beginWord and endWord), and a dictionary’s word list, find the length of shortest transformation sequence from beginWord to endWord.
(词语阶梯)
Note:
- Only one letter can be changed at a time.
- Each transformed word must exist in the word list. Note that beginWord is not a transformed word.
- Return 0 if there is no such transformation sequence.
- All words have the same length.
- All words contain only lowercase alphabetic characters.
- You may assume no duplicates in the word list.
- You may assume beginWord and endWord are non-empty and are not the same.
Example:
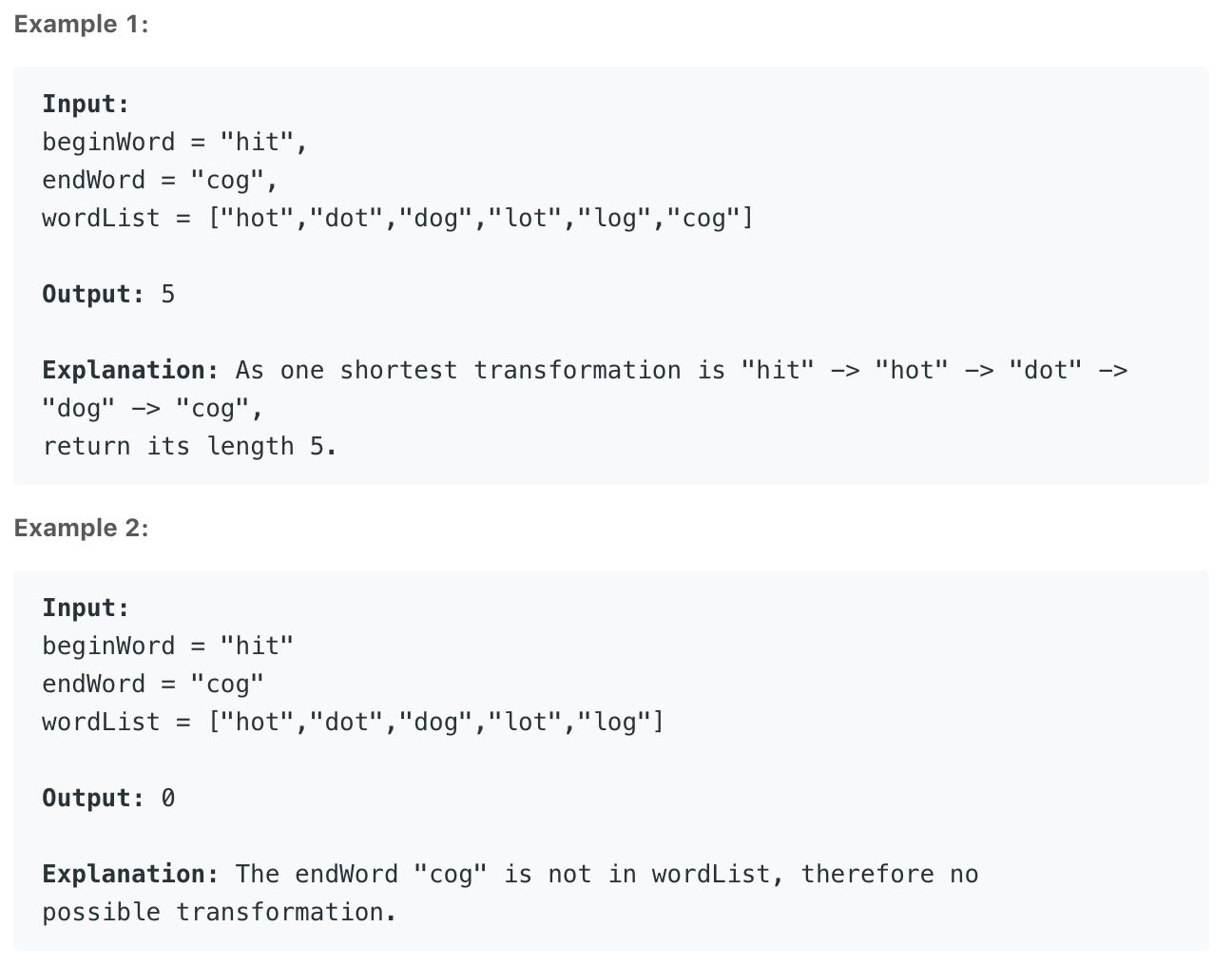
1. 宽度优先搜索
根据题意实际上是找到从 beginWord 转换到 endWord 总共需要的步数。首先根据处理 wordList 将可以通过一次相互转换的 word 放入一个map中,根据bfs的过程遍历word所有可能的转换(经过转换的词语需要标记为visited)。具体实现过程如下:
1 | class Solution: |
Note: 这里如果不事先处理 wordList 而是用 a-z 替换 word 中的字符回导致超时。