Single Number
Given a non-empty array of integers, every element appears twice except for one. Find that single one.
(只出现一次的数字(其余出现两次))
Note: Your algorithm should have a linear runtime complexity. Could you implement it without using extra memory?
Example:
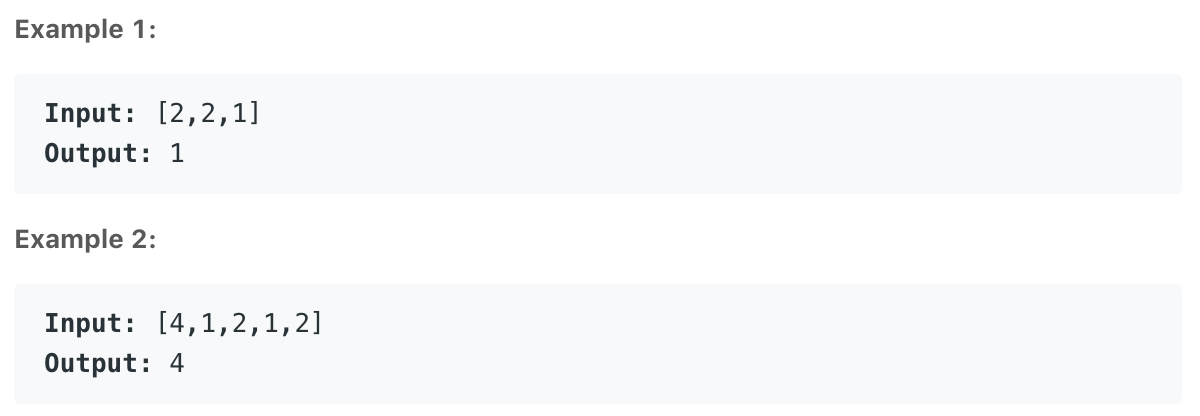
1. 哈希表
使用哈希表保存数组,时间复杂度为O(n),空间复杂度为O(n)。具体实现过程如下:
1 | class Solution: |
2. 按位异或
题中要求使用时间复杂度为O(n),空间复杂度为O(1)。在遍历数组的过程中使用按位异或的方法,最终会保留下只存在一次的数字。具体实现过程如下:
1 | class Solution: |