Insert in Sort List
Sort a linked list using insertion sort.
(基于链表的插入排序)
Example:
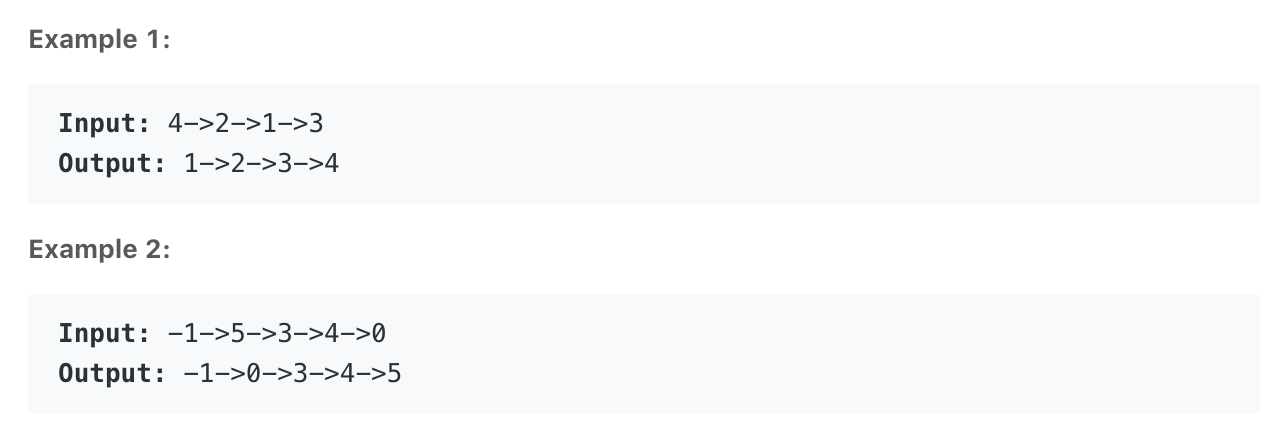
1. 头指针
维护一个含头指针的链表 j 来存储排序之后的结果,在遍历链表 i 的过程中,不断地扫描定位到链表 j 的位置,然后插入。具体实现过程如下:
1 | # Definition for singly-linked list. |
Sort a linked list using insertion sort.
(基于链表的插入排序)
Example:
维护一个含头指针的链表 j 来存储排序之后的结果,在遍历链表 i 的过程中,不断地扫描定位到链表 j 的位置,然后插入。具体实现过程如下:
1 | # Definition for singly-linked list. |