LRU Cache
Design and implement a data structure for Least Recently Used (LRU) cache. It should support the following operations: get and put.
- get(key) - Get the value (will always be positive) of the key if the key exists in the cache, otherwise return -1.
- put(key, value) - Set or insert the value if the key is not already present. When the cache reached its capacity, it should invalidate the least recently used item before inserting a new item.
(设计LRU算法的数据结构)
Follow up: Could you do both operations in O(1) time complexity?
Example:
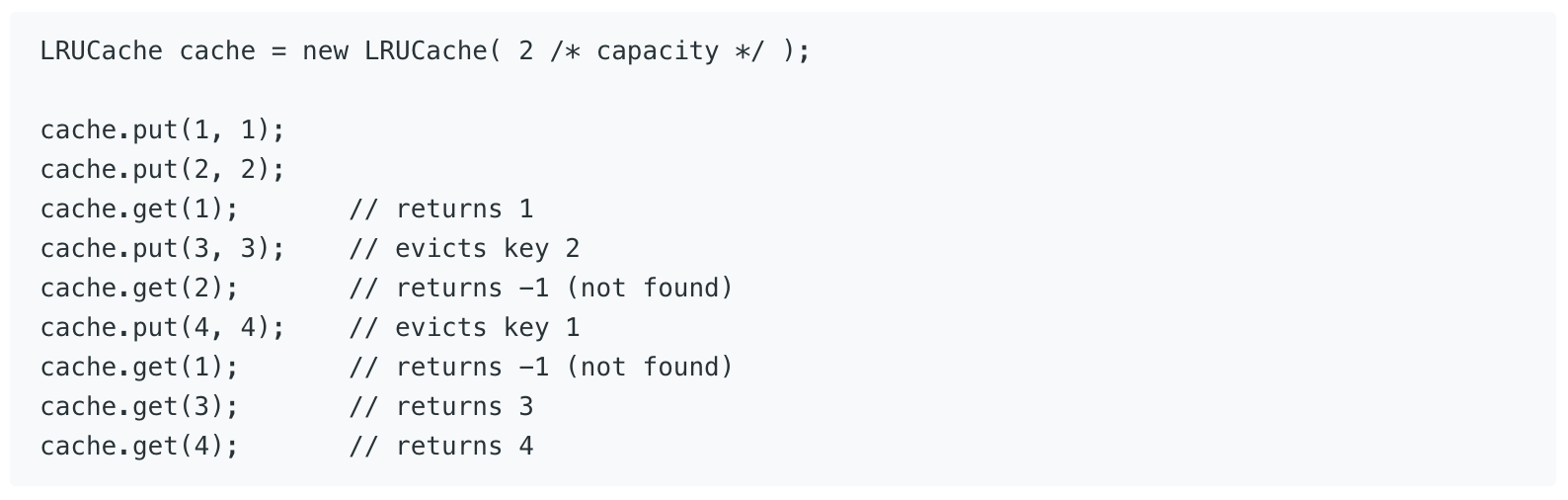
1. 哈希表 + 双向链表
这里使用哈希表和双向链表来构造 LRU 算法的数据结构,哈希表根据 key 可以直接索引到链表中相应的节点,可以实现时间复杂度是 O(1)。具体实现方法如下:
Note:
- 在进行 get/put 操作之后,需要重新将该节点移到队首。
- 双向链表方便进行队首插入新节点,队尾删除纠结点的操作。
1 | class Node: |