Max Points on a Line
Given n points on a 2D plane, find the maximum number of points that lie on the same straight line.
(坐标系中共线最多的点数)
Example:
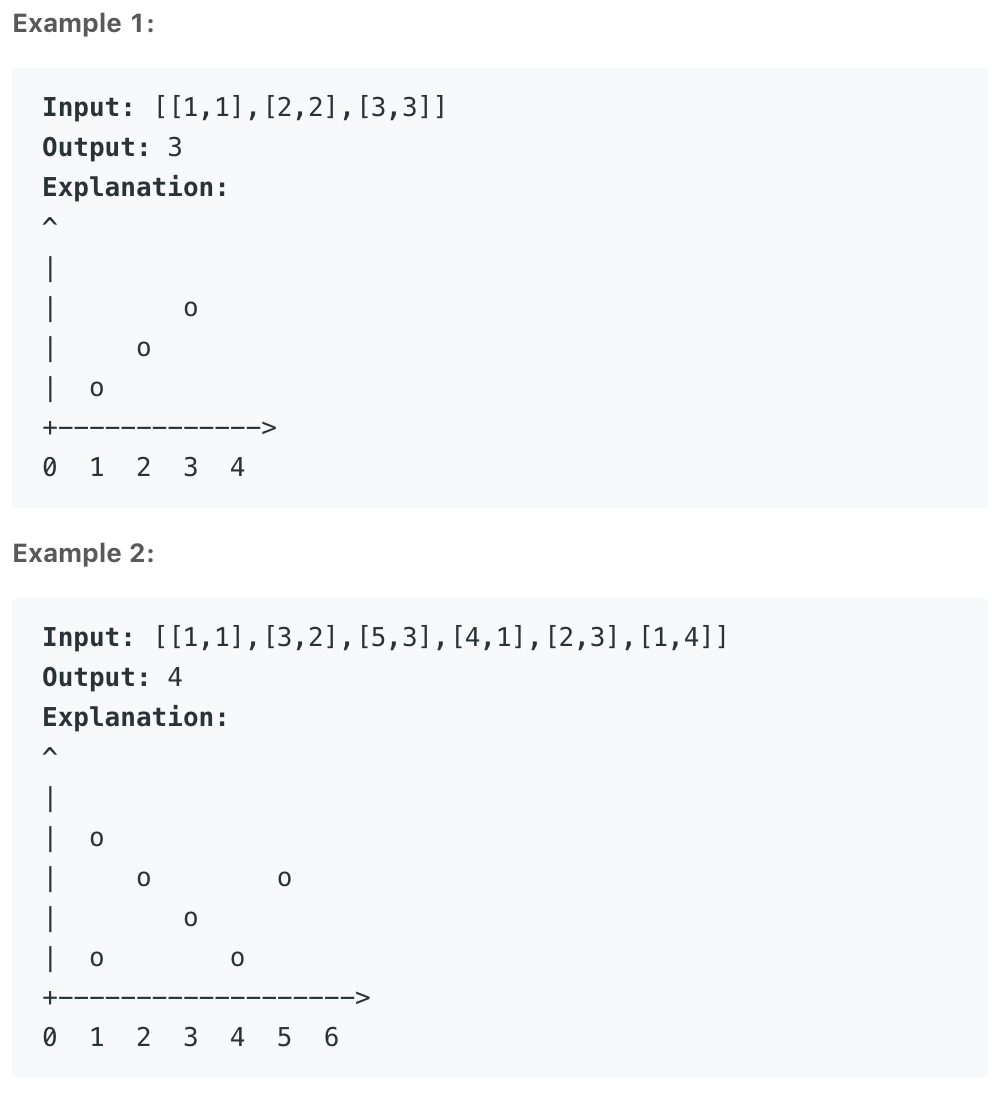
1. 哈希表 + 穷举
对每个点都计算一下该点和其他点连线的斜率,这样对于这个点来说,相同斜率的直线有多少条,就意味着有多少个点在同一条直线上。用哈希表,以斜率为key,记录有多少重复直线。但是通过斜率来判断共线需要用到除法,而用double表示的双精度小数在有的系统里不一定准确,为了更加精确无误的计算共线,我们应当避免除法,而使用约分之后的分数表示(记录分子分母)。具体实现过程如下:
1 | # Definition for a point. |