Reorder List
Given a singly linked list L: L0→L1→…→Ln-1→Ln, reorder it to: L0→Ln→L1→Ln-1→L2→Ln-2→… You may not modify the values in the list’s nodes, only nodes itself may be changed.
(链表重新排序)
Example:
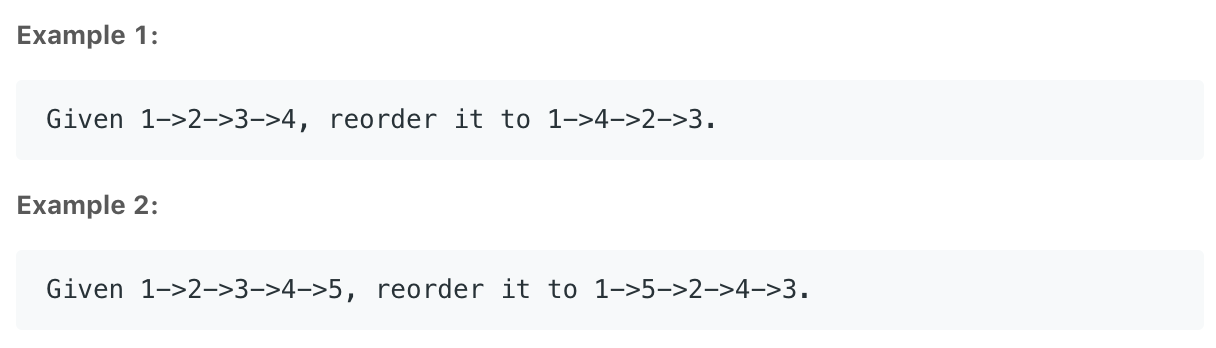
1. 切分 & 倒叙链表
首先使用 slow 和 fast 两个指针来遍历链表,找到链表的中部;然后将链表后半部分倒序;最终将倒序后的后半部分的链表插入到前半部分中。时间复杂度为O(n),时间复杂度为O(1)。具体实现方法如下:
1 | # Definition for singly-linked list. |