Maximum Gap
Given an unsorted array, find the maximum difference between the successive elements in its sorted form. Return 0 if the array contains less than 2 elements.
(乱序数组的排序后相邻元素差最大值)
Example:
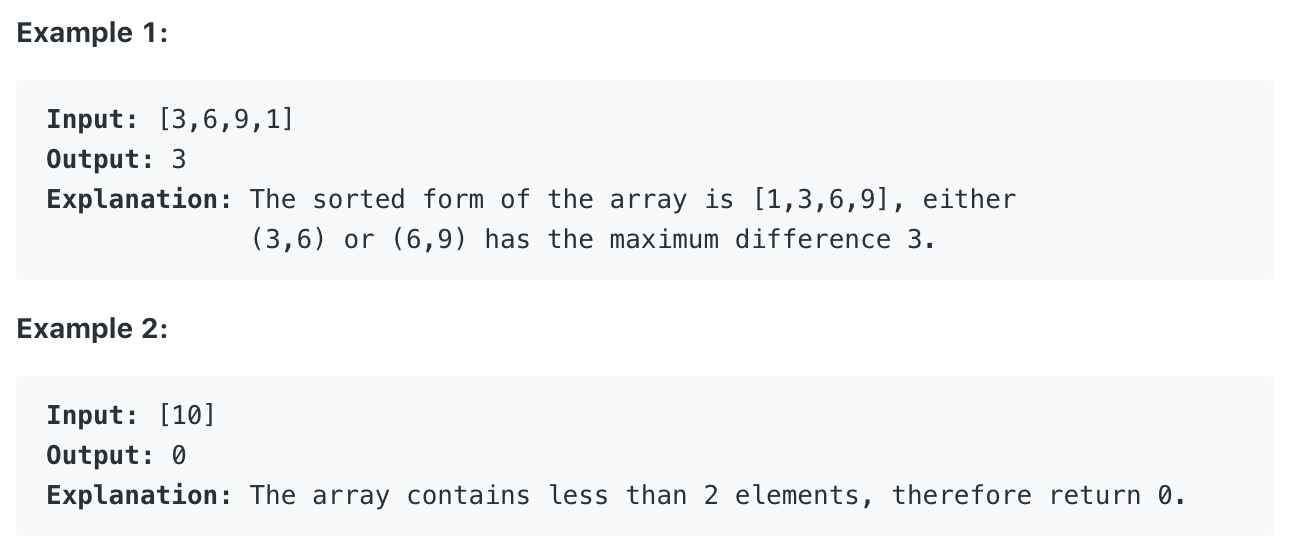
1. 基数排序
这道题的本质是一个排序问题,要求在时间复杂度和空间复杂度都是 O(n) 的前提下完成任务。基数排序的时间复杂度为 O(d*(n+k))≈O(n),空间复杂度是 O(n+k)≈O(n),其中 k 为基数,d为在k进制里面的最大位数。具体实现过程如下:
1 | class Solution(object): |