Two Sum II
Given an array of integers that is already sorted in ascending order, find two numbers such that they add up to a specific target number. The function twoSum should return indices of the two numbers such that they add up to the target, where index1 must be less than index2.
(一个升序数组中某两个元素的和为给定值)
Note:
- Your returned answers (both index1 and index2) are not zero-based.
- You may assume that each input would have exactly one solution and you may not use the same element twice.
Example:
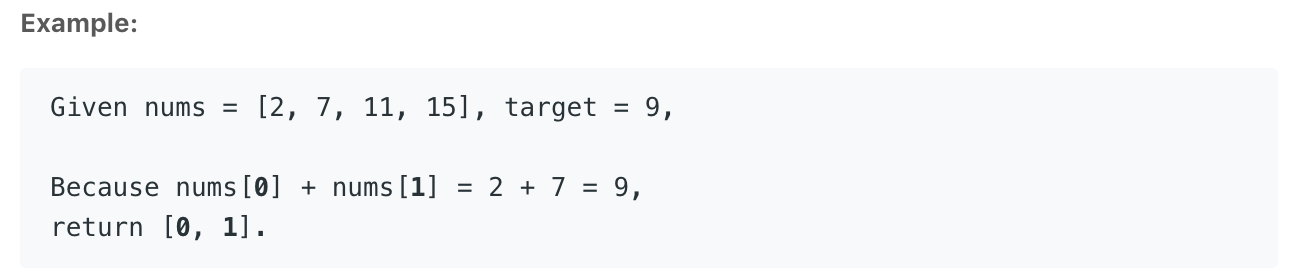
1. 双指针
首尾指针指向数组,通过与 target
判断大小来移动左、右指针,具体实现过程如下:
1 | class Solution: |
2. 二分查找
首先定位左边的元素,然后通过二分查找查找右边的元素,具体实现过程如下:
1 | class Solution: |