Dungeon Game
The demons had captured the princess (P) and imprisoned her in the bottom-right corner of a dungeon. The dungeon consists of M x N rooms laid out in a 2D grid. Our valiant knight (K) was initially positioned in the top-left room and must fight his way through the dungeon to rescue the princess.
(勇士解救公主需要的最低能量)
In order to reach the princess as quickly as possible, the knight decides to move only rightward or downward in each step. Write a function to determine the knight’s minimum initial health so that he is able to rescue the princess.
Example:
For example, given the dungeon below, the initial health of the knight must be at least 7 if he follows the optimal path RIGHT-> RIGHT -> DOWN -> DOWN
.
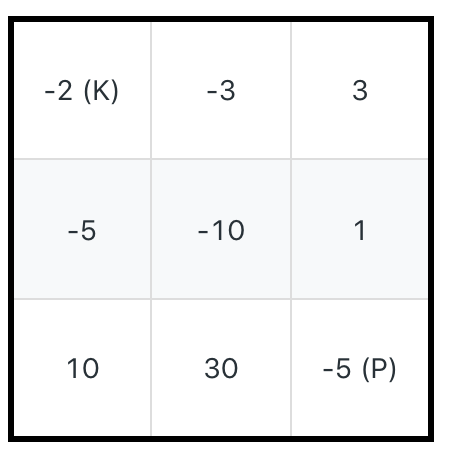
1. 动态规划
为了保证勇士能够顺利到达公主的位置,所以每个位置的最低能量值都是1。从矩阵的右下角出发不断往回回溯,需要每个方格需要的最小能量值。具体的动态规划递推表达式如下:
1 | class Solution: |