Majority Element
Given an array of size n, find the majority element. The majority element is the element that appears more than ⌊ n/2 ⌋ times. You may assume that the array is non-empty and the majority element always exist in the array.
(众数(次数大于n/2))
Example:
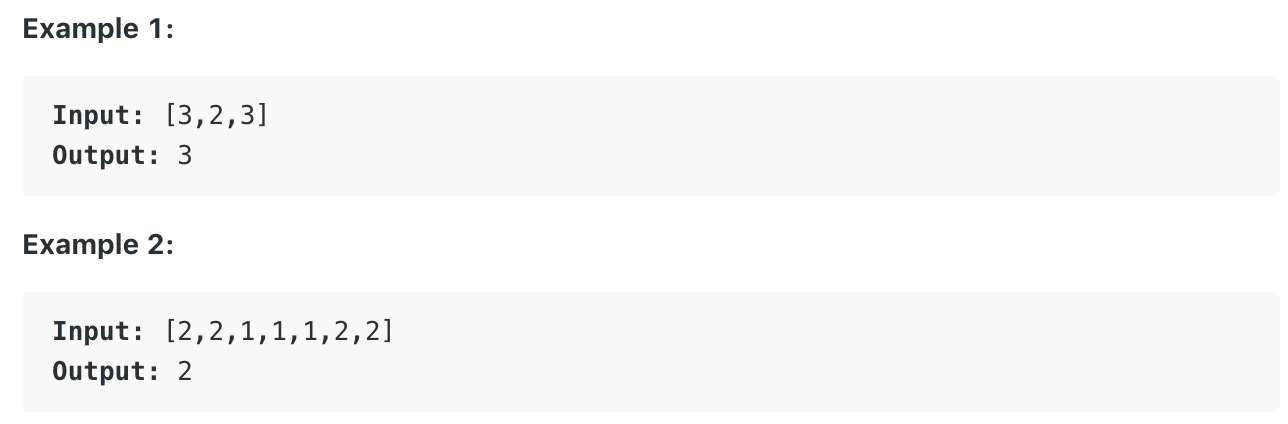
1. 哈希表
构建一个哈希表来保存数组,统计每个元素的个数。也就是实现函数collections.Counter(nums)
的功能。具体实现过程如下:
1 | from collections import defaultdict, Counter |
2. 排序
由于此处的众数的定义为出现频次超过 n/2 的元素,因此先将数组进行排序,然后第 n/2 个元素一点就是“众数”。具体实现过程如下:
1 | class Solution: |