Rotate Array
Given an array, rotate the array to the right by k steps, where k is non-negative.
(翻转数组)
Note:
- Try to come up as many solutions as you can, there are at least 3 different ways to solve this problem.
- Could you do it in-place with O(1) extra space?
Example:
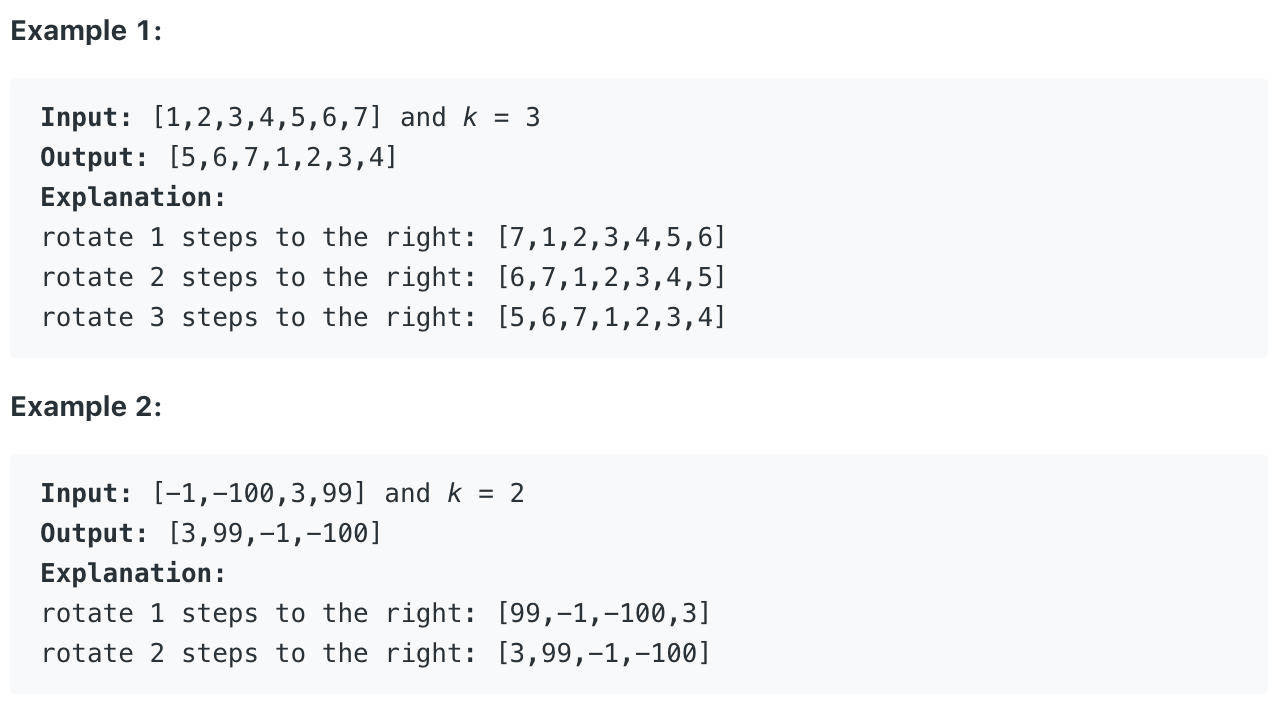
1. 数组拼接
根据上面的例子我们可以总结出如下规律,在经历了 k 次旋转之后的数组为原始数组的 [n-k:] 和 [:n-k] 两个数组拼接在一起的结果。其时间复杂度为 O(n) ,空间复杂度为 O(1) 。具体实现过程如下:
1 | class Solution: |
2. 3次旋转数组
根据上面的例子我们可以总结出如下规律,在经历了 k 次旋转之后的数组为 1-原始数组全部旋转,2-前k项旋转,3-后n-k项旋转的结果。其时间复杂度为 O(n) ,空间复杂度为 O(1) 。具体实现过程如下:
1 | class Solution: |