Course Schedule II
There are a total of n courses you have to take, labeled from 0 to n-1. Some courses may have prerequisites, for example to take course 0 you have to first take course 1, which is expressed as a pair: [0,1]. Given the total number of courses and a list of prerequisite pairs, return the ordering of courses you should take to finish all courses. There may be multiple correct orders, you just need to return one of them. If it is impossible to finish all courses, return an empty array.
(课程清单,给出具体的结果)
Example:
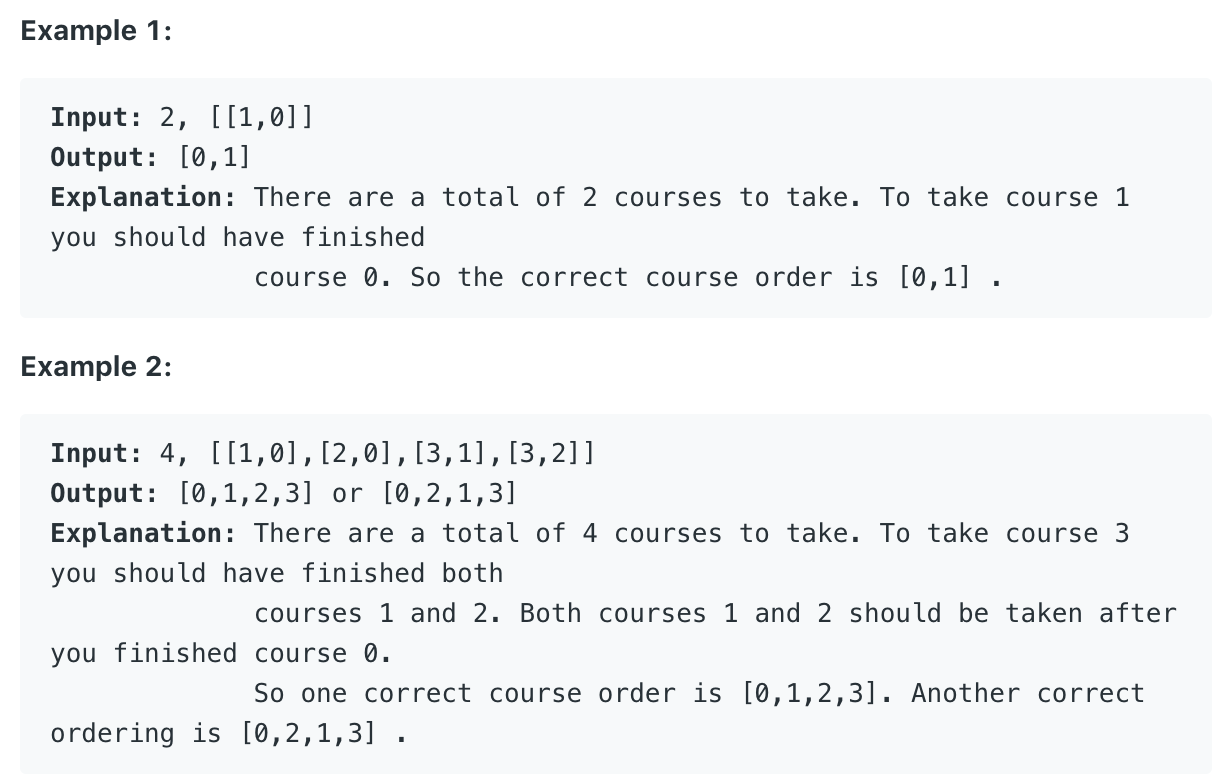
1. 拓扑排序-BFS
根据 “Course Schedule” 的步骤可以得出具体的结果,时间复杂度为O(n),空间复杂度为O(n)。具体实现过程如下:
1 | import collections |
2. 拓扑排序-DFS
同样地,时间复杂度为O(n),空间复杂度为O(n)。具体实现过程如下:
1 | class Solution: |