Convert Binary Search Tree to Sorted Doubly Linked List
Convert a BST to a sorted circular doubly-linked list in-place. Think of the left and right pointers as synonymous to the previous and next pointers in a doubly-linked list.
(将二叉搜索树转换为一个有序的双向链表)
Example:
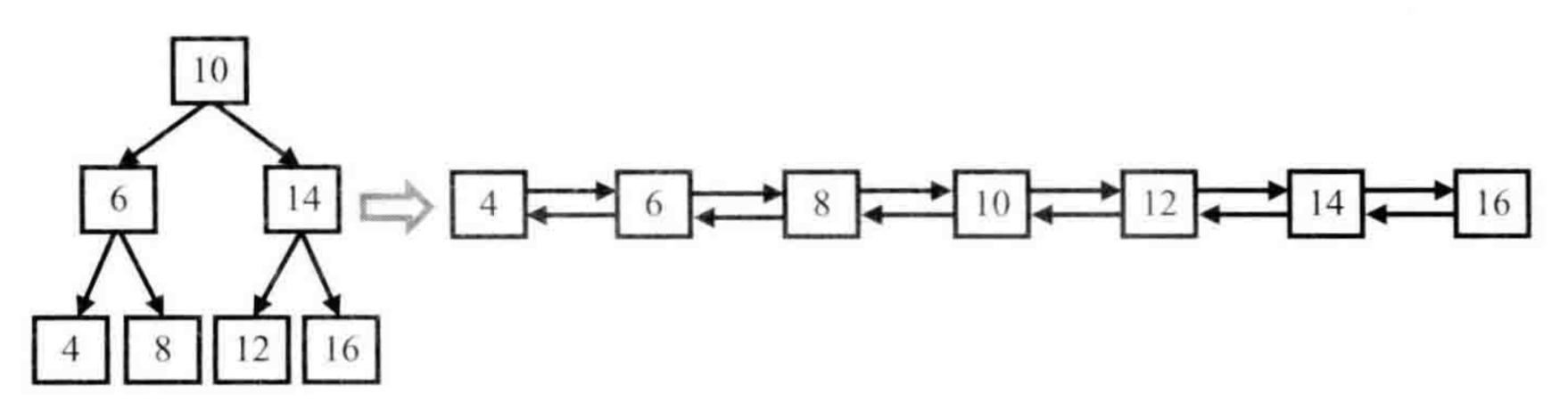
1. 中序遍历
首先对二叉树进行中序遍历,将遍历后的结果进行指针调整,时间复杂度为O(n),空间复杂度为O(n)。具体实现过程如下:
1 | # class TreeNode: |
2. 中序遍历
在对二叉树进行中序遍历时进行指针调整,时间复杂度为O(n),空间复杂度为O(1)。具体实现过程如下:
1 | # -*- coding:utf-8 -*- |